What are command line arguments in python?
In the command line, we can start a program with additional arguments.
These arguments are passed into the program.
Python programs can start with command line arguments. For example:
where program.py and image.bmp is are arguments. (the program is Python)
Related Course:
Complete Python Programming Course & Exercises
How to use command line arguments in python?
We can use modules to get arguments.
Which modules can get command line arguments?
Module | Use | Python version |
---|---|---|
sys | All arguments in sys.argv (basic) | All |
argparse | Build a command line interface | >= 2.3 |
docopt | Create command line interfaces | >= 2.5 |
fire | Automatically generating command line interfaces (CLIs) | All |
optparse | Deprecated | < 2.7 |
Sys argv
You can get access to the command line parameters using the sys module. len(sys.argv) contains the number of arguments. To print all of the arguments simply execute str(sys.argv)#!/usr/bin/python
import sys
print('Arguments:', len(sys.argv))
print('List:', str(sys.argv))
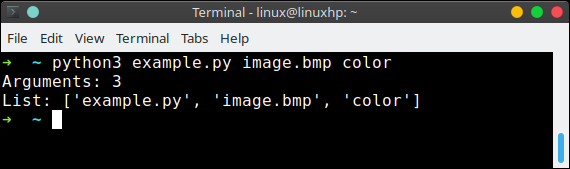
python sys argv example
You can store the arguments given at the start of the program in variables.
For example, an image loader program may start like this:
#!/usr/bin/python |
Another example:
(‘List:’, “[‘example.py’, ‘world.png’]”)
(‘Filename:’, ‘world.png’)
Related Course: Complete Python Programming Course & Exercises
Argparse
If you need more advanced parsing, you can use argparse.
You can define arguments like (-o, -s).
The example below parses parameters:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('-o','--open-file', help='Description', required=False)
parser.add_argument('-s','--save-file', help='Description', required=False)
args = parser.parse_args()
print(args.open_file)
print(args.save_file)
Docopt
Docopt can be used to create command line interfaces.
|
**Note:** docopt is not tested with Python 3.6
Fire
Python Fire automatically generates a command line interface, you only need one line of code. Unlike the other modules, this works instantly.
You don’t need to define any arguments, all methods are linked by default.
To install it type:
Then define or use a class:
|
python example.py openfile filename.txt
Why is bmp is an argument , in my computer only a .py extensional file came up ?
I write a bmp filename in the command, this is the only reason. By default only .py files should come up.
There is two issue with second example code:
NOT like this:1. In line # 9: it should be like this:
2. Line #12 & 13 should be in else statement like this:
thanks.