The argsparse module can be used to parse command line arguments.
This article is written for argsparse with Python 3.
Sure you could write your own parser to get command line arguments from sys.argv, but argparse makes it easier.
By default it will add a help option, even if you don’t specify it. Let’s show the sort of thing you can do with argsparse.
Related Course: Complete Python Programming Course & Exercises
Arguments
The code below is a Python program that takes opitional arguments. We create an ArgumentParser instance and create new arguments by callig nt he method add_argument().
Then when the method parse_args() is called, it uses all that information and interprets sys.argv automatically!
import argparse |
If we save the code above as example.py, it can be run like this:
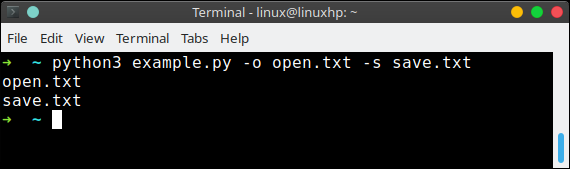
We have set the parameter required=False, they are optional.
open.txt
If we set the last parameter to required=True, it has to be specified or it will throw an error.