A dictionary dict in Python is a one to one mapping, it contains a set of (key,value) pairs where each key is mapped to a value. It’s an example of a hash map or hash table (from Computer Science).
Every key points to a value, separated by a colon (:).
A dictionary is defined using curly brackets. The value left of the colon is called the key, the value right of the colon is called the value. Every (key,value) pair is separated by a comma.
Related Course: Complete Python Programming Course & Exercises
Python Dictionaries or Hashmaps
A dictionary can store objects (ints, floats, strings). Each object (key) is mapped to another object (value). Sometimes they are called maps, hashmaps, associative arrays or lookup tables.
In the real world, a phone book is an example of a dictionary. You can quickly get a phone number, by searching through the list of keys (the persons name).
A core idea is that you can quickly get a value using a dictionary, without having to use a (for) loop.
To keep with the previous example of phone books, a dictionary can be made like this:
phoneBook = { |
The names are the keys, and each of them is mapped to a value. Such that ‘alice’ is mapped to 1947, ‘bob’ is mapped to ‘9114’ and so on.
You can then retrieve information by using its key:
'jack'] phoneBook[ |
You can create a dictionary one a single line (if you want to do so). But keys must be unique values, you can not use the same key twice. Values may or may not be unique.
k = { 'en':'English', 'fr':'French' } |
We defined a dictionary named k and access elements using the square brackets you’ve seen before. We use the key['en']
to print the value ‘English’.
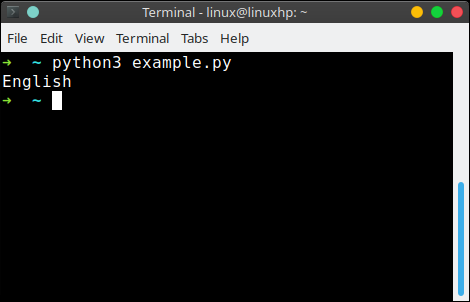
Note: A dictionary has no specific order.
Related Course: Complete Python Programming Course & Exercises
Dictionary append
A dictionary is not a constant, it can be changed during execution.
If you have an existing dictionary, you can add new (key,value) pairs to it.
phoneBook = { |
To add a new value to a dictionary you can simply assign a key value pair:'rosa'] = 3524 phoneBook[
Dictionary remove
Do you want to remove a (key,value) pair from a dictionary? No problem.
To remove a (key,value) pair from a dictionary you can use the del keyword followed by the key:
del phoneBook['rosa'] |
The example below shows a complete example of adding and removing in the Python interactive shell:
phoneBook = { |
Built-in dict type
The default type for dictionaries in Python is dict
. You can verify this by typing type(dict)
.
A small example in the shell:
phoneBook = { |
Python’s built-in dictionary implementation will do everything you need for most use cases. However alternative implementations exist.
Related Course: Complete Python Programming Course & Exercises
collections.OrderedDict
OrderedDict is an alternative dictionary implementation, its part of the collections
module. collections.OrderedDict
remembers the order of insertion. For example:
import collections |
This shows the (key,value) pairs are in the same order as you added them. With the Python standard dictionary that is not always the case.
You can also get its keys:
phoneBook.keys() |
collections.ChainMap
ChainMap is yet another implementation. It lets you search multiple dictionaries as if it were one.
It’s part of the collections
module, to load it use:
from collections import ChainMap |
You can define several dictionaries and create a ChainMap. Then you can use it as if were one single dictionary.
The example below combines two dictonaries (phoneBook1, phoneBook2) and creates a ChainMap. Then it loads (key,value) pairs that were originally in one of the two dictonaries.
from collections import ChainMap |
If you are a Python beginner, then I highly recommend this book.