If you aim to optimize your code for reusability, Python functions are indispensable.
Functions allow you to execute specific blocks of code multiple times without redundancy.
In programming, giving each function a unique name is crucial for its identification. When you invoke this name, it prompts the execution of a predefined code block associated with it.
**Related Course: ** Complete Python Programming Course & Exercises
Understanding Python Functions
Functions are fundamental building blocks in coding. They partition your code into specific segments or modules. This structured approach enhances code clarity and simplifies debugging. An everyday function in Python you might have encountered is the print function:
print("Hello world") |
The beauty of functions is their reusability. You can call the same function multiple times:
print("Coffee") |
Creating a Python Function
To define a function in Python, adhere to the following syntax:
def <function_name>([<parameters>]): |
Here’s a breakdown:
def
signals Python that you’re defining a function.function_name
is the name of your function and should be unique in your code.parameters
are optional inputs that your function can utilize.:
concludes the function header.<statement(s)>
are the actions or commands within the function, also known as the function’s body. Always remember to indent them by four spaces.
Let’s see a simple function f()
in action:
def f(): |
Given that functions are reusable, invoking it multiple times is straightforward:
f() |
Your function’s body can also span several lines:
def hello(): |
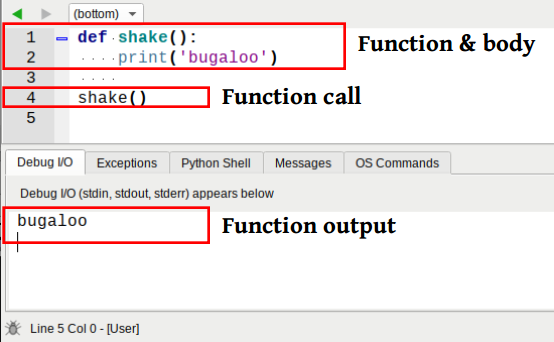
If you are a Python beginner, then I highly recommend this book.
Function Parameters and Arguments
Parameters are the input values that functions can accept. Always ensure they maintain their order when used:
def hello(name): |
To define multiple parameters, separate them with commas:
def f(name, salary): |
When calling a function, always ensure that the arguments match the order and number set in the function’s definition. The pass
keyword in Python denotes a function with no operations.
Utilizing Default Parameters
Default parameters provide values to a function when no arguments are supplied. These values act as fallbacks:
def f(x=5,y=4): |
Understanding Function Scope
Variables declared within a function remain constrained to that function. They’re inaccessible outside its boundaries. To make a function’s internal variable available outside, employ the return
keyword.
The Power of the Return Statement
Using return
, functions can produce outputs which can then be stored in variables for further use:
def sum(a,b): |
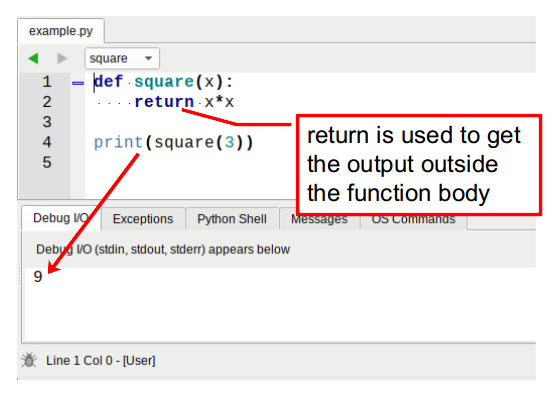
Functions in Python are flexible enough to return multiple values simultaneously:
def f(a,b,c): |
Handling Variable-Length Arguments
Sometimes, the number of arguments a function should accept can be variable. To accommodate this, use lists or tuples:
def f(*args): |
Embracing Docstrings
Docstrings offer a documentation mechanism for functions, helping users understand their purpose and usage. Always strive to document complex or non-intuitive parts of your code.
The Significance of the main() Function
The main()
function, commonly used in Python scripts, serves as the program’s entry point. It’s especially useful for scripts meant to be both standalone and importable modules.
Summing Up
Functions are an integral part of Python programming, offering structure and reusability. In this guide, you discovered the ins and outs of Python functions, from their creation and invocation to advanced features like default parameters and docstrings.
If you are a Python beginner, then I highly recommend this book.
You got a small typo below the first block of code. I think it's supposed to be 'y=3'. :)
Thanks Sebastian!
How do you do addition and subtraction?
By the way I am a beginner
When I initially commented I clicked the "Notify me when new comments are added" checkbox and now each time a comment is added I get three e-mails with the same comment. Is there any way you can remove people from that service? Cheers!
Removed your mail address, should be good now. Let me know if you still get mails