What is list comprehension?
List comprehensions are an easy way to create lists (based on existing lists).
Its much easier to write a one liner than it is to write a for loop, just for creating a list. This _ one liner_ is called a list comprehension.
Related Course: Complete Python Programming Course & Exercises
Introduction
In Python, the syntax of list comprehension is:
list_variable = [x for x in iterable]
Assume we want to create a list containing 100 numbers. Manually that would be a lot of typing work. So we would use a for loop, right?
We can define a for loop to fill the list.numbers = []
for i in range(0,100):
numbers.append(i)
print(numbers)
That works, but it’s to much work. Instead you can use list comprehension.
You can replace all the code above with a one liner, which is how we obtain the same result:numbers = [ x for x in range(100) ]
yes, inside the list we use a loop and the range() function
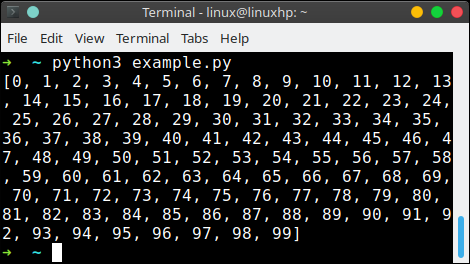
This is also useful if you want to create large lists.
List Comprehensions
Assume we want all the square roots to 100:import math
numbers = [ math.sqrt(x) for x in range(100) ]
print(numbers)
You can also use it like this:
for letter in 'hello' ] h = [ letter |
An if statement can be inside a list comprehension
for x in range(20) if x % 2 == 0] number_list = [ x |
It can be used on a list of strings too:
'alice','xena','bob','veronica' ] player_list = [ |