Make a graphical interface with PyQt?
Graphical interfaces made with PyQt run on: Microsoft Windows, Apple Mac OS X and Linux. We will create a Hello World app with PyQt.
Related course: Create PyQt Desktop Appications with Python (GUI)
PyQt installation
PyQt is available for both Python 2 (2.7.9 tested) and Python 3.
To install write:
pip3 install pyqt5
With apt-get you can use: python3-pyqt5
PyQt hello world
The app we write will show the message “Hello World” in a graphical window. Import the PyQt5 module.
# https://pythonprogramminglanguage.com/pyqt5-hello-world/ |
The program starts in the main part.
We initialize Qt and create an object of the type HelloWindow.
We call the show() method to display the window.
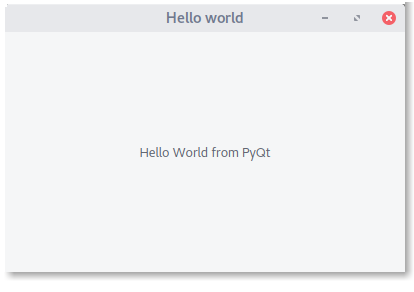
if __name__ == "__main__": |
The HelloWindow class inherits from the class QMainWindow.
We call its super method to initialize the window.
Several class variables are set: size and window title.
We add widgets to the window, including a label widget (QLabel) which displays the message “Hello World”.
If you are new to Python PyQt, then I highly recommend this book.