What is the difference between global and local variables?
A global variable can be accessed anywhere in code. A local variable can only be used in a specific code block.
Related course: Complete Python Programming Course & Exercises
global variable example
We say the scope is global, it can be used in any function or location throughout the code.
In the example below we define a global variable, y amount lines of code later we can still use that variable.
x = 3
...
200 lines of code
...
print(x)
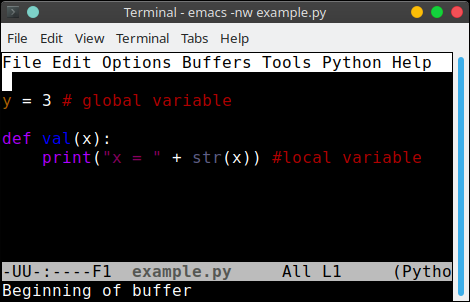
local variables
This is contrary to a local variable, which can only be accessed in the local scope.
In the example below, x is a local variable..
def f(x):
.. x can only be used here
If a variable is declared inside a function or loop, it’s a local variable. Global variables are usually defined at the top of the code.
best practice
Global variables are considered a bad practice because functions can have non-obvious behavior. Usually you want to keep functions small, staying within the size of the screen. If variables are declared everywhere throughout the code, it becomes less obvious to what the function does.
If you are a Python beginner, then I highly recommend this book.