Variables serve as a foundational concept in programming. In this guide, we delve deep into the nature and usage of Python variables, showing the distinction between constant numbers or literals.
print(3.3) |
The code above shows a direct usage of a number. However, when we build more complex applications, we inevitably rely on variables.
Master Python: Complete Python Programming Course & Exercises
How to Assign Variables in Python
Imagine a variable as a labeled box where you store a value. The label is the variable name and the content is the value. The assignment process uses the equals sign (=
).
x = 3 |
This can be interpreted as ‘x holds the value 3’. After this assignment, you can refer to x
throughout your program.
print(x) |
Using the interactive Python shell (REPL), you can also retrieve its value directly.
x |
Python allows you to update the value of x
or any variable as your program progresses.
x = 4 |
When we retrieve x
again, we observe the updated value.
print(x) |
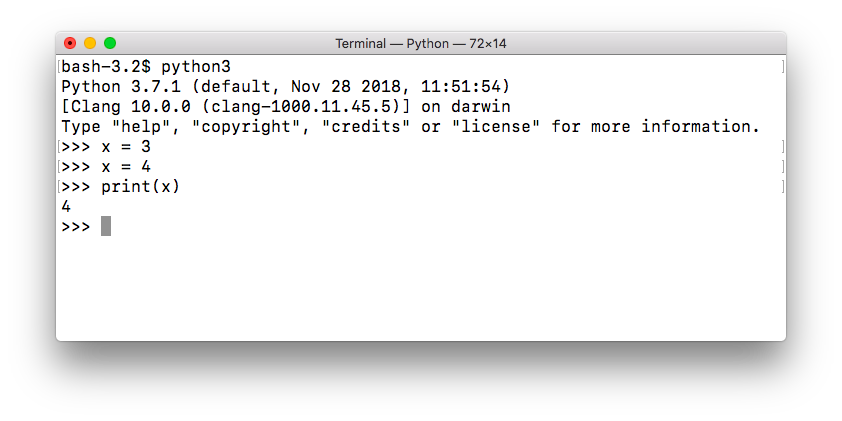
Deep Dive into Python: Complete Python Programming Course & Exercises
Exploring Variable Types in Python
A remarkable feature of Python is its dynamic typing system. Unlike some languages that mandate prior type declarations (statically typed), Python infers the type of a variable at runtime.
"hello" y = |
Notice how the type of y
transitions? You can inspect a variable’s type with the type()
function.
type(y) |
Here, y
changes from a str
(string) to an int
(integer).
str
: Represents textual content, which can range from a single character to entire paragraphs.int
: Denotes whole numbers, e.g., 1, 2, 3.
Python’s dynamic typing means the language determines the most appropriate type.In the above code, the variables x, price, and word are assigned different types.3 x =
5.50 price =
"hello" word =Here,type(x)
<class 'int'>
type(price)
<class 'float'>
type(word)
<class 'str'>float
represents numbers with decimal points.
Advance Your Python Skills: Complete Python Programming Course & Exercises
Guidelines for Naming Variables
While we’ve seen brief variable names like x and y, Python supports long, descriptive names comprising uppercase and lowercase letters, underscores, and numbers. But remember, they can’t include spaces or special characters.
"Alice" name = |
However, initiating a variable name with a number is invalid.
In Python, variable names are case-sensitive. Thus, name
, Name
, and NAME
are distinct.
Explicit naming, which makes the code self-explanatory, is preferable. For instance, using camel casing:
"Bob" NameOfFriend = |
Naming conventions include:
- Camel Case:
MyVariableName
- Snake Case:
my_variable_name
- Pascal Case:
MyVariableName
The style chosen often depends on personal or organizational preference. Notably, camel case is widely adopted in languages like Java and C++.
Words Reserved by Python
Certain words are reserved by Python, meaning they cannot be used as variable names. To view these, use help("keywords")
.
help("keywords") |
Strings in Python
Strings, which hold textual content, can be defined in three different ways:
word = 'Hello world' |
Though all three declarations are valid, double quotes are most commonly used.
Mathematical Operators in Python
Once variables are assigned, they can be utilized and manipulated across the program. Python supports standard arithmetic operators.
x = 2 |
Utilizing Formatted Strings in Python
Variables can be displayed using the print()
function. To embed variables within textual content, we use formatted strings.
x = 5 |
Formatted strings provide a neat way to interleave variables and text for clearer output.
Pursue Mastery in Python: If you are a Python beginner, then I highly recommend this book.
Next Steps in Python Journey: Download exercises
As stated above the 3 ways to define text variables, may I know the differences among them? As in Java single quotes used for single character where in double quotes is used for strings
All methods can be used to define characters or strings. In Java there is an explicit difference between single and double quotes, but in Python any form can be used for both strings and single characers. In Python it's simply a matter of preference.
So, there's no need to instantiate integers or other variables?
Exactly, this is optional.
what is the use of str() function?why we should define +str()?
str() converts a number to (printable) text.
I suppose that by "we have tree variables" you meant "we have three variables"
Thanks, it's corrected
>>> x=5
>>> print("x = " + str(x))
Output: x = 5 its not getting printed as string
Run the code from a file (program.py)
1. save as text file (program.py)
2. python program.py
What does the command 'print("x="+str(x))' print ?
It prints the value inside x and a text description: "x=.."