You can create a chain of if statements using the keywords elif and else. This is a lot easier to read than having to read ‘if if if’ all over again.
So using the keywords elif and else. That way, we can walk through all options for the condition. Imagine a weather app: if snow, elif rain, elif thunder, else sunny. We can execute different code for each condition.
Related Course: Complete Python Programming Course & Exercises
If statement
Recall that you can create a branch in your code using an if statement, which could be one single code line or a block of code.
if <condition>: |
Instead of block of code, we say branch.
This creates a branch that is executed based on the condition. If the condition is True, the code is executed. If the condition is False, the code is skipped.
Each branch is indented with 4 spaces.
How to use “else condition”
So what if the <condition>
is not True?
The program continues execution.
if <condition>: |
If you want to add a False check, you can add else
.
if <condition>: |
if <condition>
is True it runs the first branch.
If <condition>
is False, it jumps into the second branch.
Related Course: Complete Python Programming Course & Exercises
If elif and else
You can also do that if we have multiple conditions. Instead of doing this:
x = 3 |
You can connect the if statements with the keyword else. The example below shows how to do that.x = 3
if x < 5:
print('x smaller than 5')
else:
print('x is too big')
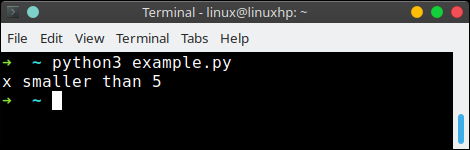
In the above example there are two branches, each branch has a single line of code (but it can be multiple).
Because the value of x is 3 x = 3
it jumps into the first branch and then exists. If you change the value of x to be greater or equal to five, it will jump in the second branch.
After completion of the if statement it will continue executing the program in the normal flow.
When “else condition” does not work
It may be that the else
doesn’t give the desired result. For instance, in this program:
x = 5 |
In this case the value of x is equal to five.
To correct this you can add an equality check (==
) by adding the elif
clause which we will talk about more in the next section.
5 x = |
How to use “elif” condition
You can test multiple conditions by using elif
.
- First define the default condition
- then add as many other test conditions you want by adding
elif
.
Each if code should be indentend with four spaces. The if codes body ends when the four spaces are removed again.
2 x = |
This can be as long as you want. You can keep adding the conditions you need.
if x > 10: |
Like the example above. You can optionally add another else
to the end of the list.
Related Course: Complete Python Programming Course & Exercises
Switch statement
Instead of having a long list of elif
, many programming languages allow you to use a switch
statement.
For instance, in Java you can do this:
switch(argument) { |
This is a lot cleaner than a long list of elif
cases. But,
Python does not have a switch statement.
Instead you can use a dictionary.
Please note that this is a trick, and a normal if statement or elif cases are preferred.
The example below defines a function with a dictionary in it. By calling the function with a parameter, it simulates a switch statement.
|
This works, but does not let you check greater than >
, smaller than <
, greater or equal >=
and other conditions.
In general it’s better to use if
statements combined with elif
and else
.
Summary
In this article we talked about else
and elif
clauses, which test other for other conditions.
if
tests the default condition (is True?)Each branch must have 4 spaces
code is only executed if a condition is True
else
tests if the default condition(s) are Falseelif
tests multiple conditionsPython does not have a switch statement, but it can be simulated
If you are a Python beginner, then I highly recommend this book.