The clipboard is a fundamental tool in our daily digital tasks, allowing us to temporarily store and transfer data between programs. In this article, we’ll explore how to access and manage the clipboard using Python’s PyQt.
With PyQt, it’s possible to create a program that not only accesses the clipboard’s content but also displays it in a user-friendly interface. So, if you’ve ever wondered how to manipulate clipboard contents using a PyQt application, this is the guide for you.
**Further Reading: ** Create PyQt Desktop Appications with Python (GUI)
Accessing Clipboard with PyQt
At the core of clipboard management in PyQt is the QClipboard
class. Through this class, developers can retrieve and display copied text. Here’s a step-by-step breakdown:
Connect to the clipboard:
QApplication.clipboard().dataChanged.connect()
Fetch the current clipboard contents:
QApplication.clipboard().text()
Below is a visual representation of how the PyQt clipboard integration looks:
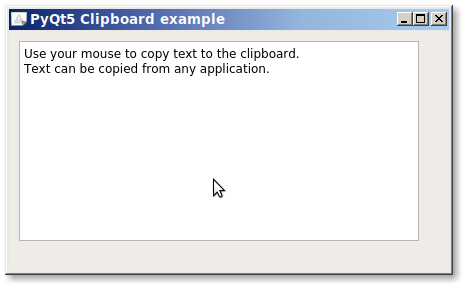
To further illustrate, let’s delve into a practical example. The following code sets up a PyQt5 application that fetches and displays text copied to the system clipboard:
import sys |
With the above code, whenever text is copied from any source, the PyQt application will instantly display it.
For more resources on PyQt and its extensive capabilities, consider exploring: If you are new to Python PyQt, then I highly recommend this book.
Happy coding, and don’t forget to dive deeper into PyQt’s extensive features! Download PyQt Examples