A text area can be created in Python PyQt with the widget QPlainTextEdit.
QPlainTextEdit is a multiline text area in PyQt. To set the text, we use its method insertPlainText(). We can set its position and size using the methods move() and resize().
If you are new to PyQt, I recommend the course below.
Related course: Create PyQt Desktop Appications with Python (GUI)
Introduction
Before you can make a window or add a text area, you should verify that you have PyQt installed and working on your computer.
The example below creates a text area QPlainTextEdit using PyQt5. You can load thie widget from PyQt5.QtWidgets
.
from PyQt5.QtWidgets import QPlainTextEdit |
You’ll also want QMainWindow and QWidget
from PyQt5.QtWidgets import QMainWindow, QWidget, QPlainTextEdit |
Then you can create a new text area widget like this:
self.b = QPlainTextEdit(self) |
You can add text into the text area programatically:
self.b.insertPlainText("You can write text here.\n") |
Set its position (horizontal,vertical) inside the window:
self.b.move(10,10) |
And turn it into the change (width,height)
self.b.resize(400,200) |
QPlainTextEdit example
We will create the usual QMainWindow to add the widget to. It is just for plain text, like notepad. To add new lines we add the \n
character.
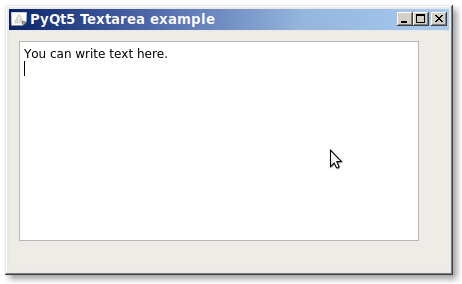
import sys |
If you are new to Python PyQt, then I highly recommend this book.
Thx!