A checkbox is often a core part of a graphical user interface, it lets a user select from one or more options which can be either True or False. It can be created using the QCheckBox widget. When creating an new checkbox with the QCheckBox class, the first parameter is the label.
To apply actions to the toggle switch, we call .stateChanged.connect() followed by a callback method. When this method is called, it sends a boolean as state parameter. If checked, its the value QtCore.Qt.checked.
Related course: Create PyQt Desktop Appications with Python (GUI)
PyQt Checkbox example
To create a checkbox, first import QCheckBox
from the module PyQt5.QtWidgets
.
#https://pythonprogramminglanguage.com/pyqt-checkbox/ |
Then create a new checkbox. As parameter you can set the text that is shown next to the checkbox.
box = QCheckBox("Awesome?",self) |
You can change its position and size, by using the objects methods .move(x,y)
and .resize(width,height)
.
box.move(20,20) |
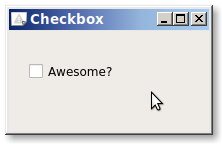
You may also want to include a callback function, that reacts to checkbox clicks
box.stateChanged.connect(self.clickBox) |
The Python PyQt example below creates a checkbox (QCheckBox
) inside a window. If you click on the checkbox, it calls the method clickBox (toggled).
#https://pythonprogramminglanguage.com/pyqt-checkbox/ |
If you are new to Python PyQt, then I highly recommend this book.