A tooltip enhances the user experience in graphical user interfaces by providing quick information about a widget. In PyQt, tooltips emerge when you hover your mouse over a widget, eliminating the need for a click. Hovering over a button, for instance, will sometimes display relevant details—this information is a tooltip.
PyQt offers comprehensive support for tooltips, allowing developers to set them up for various widgets. Additionally, tooltips in PyQt can be a mix of plain text and HTML-formatted content.
** Related Course: ** Create PyQt Desktop Appications with Python (GUI)
Setting up Tooltips in PyQt5
Note: Remember, tooltips aren’t visible on mobile devices since they lack a mouse cursor.
The setToolTip
method is essential for defining a tooltip in PyQt. Once a widget is created, this method can be employed to assign a tooltip to it.
pybutton.setToolTip('This is a tooltip for the QPushButton widget') |
To view the tooltip, hover the mouse over the widget and wait momentarily.
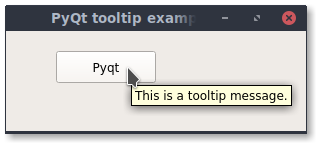
Interestingly, PyQt tooltips can contain HTML content. HTML allows for formatting using tags like <tag></tag>
.
button.setToolTip('This is a <b>QPushButton</b> widget') |
If you’re looking to tweak the tooltip’s font style, PyQt’s got you covered:
QToolTip.setFont(QFont('SansSerif', 10)) |
Practical Tooltip Example with PyQt5
This example illustrates the creation of a button in a PyQt window. The button is mapped to the clickMethod()
function—clicking the button activates this function.
Additionally, the button features a tooltip that appears when hovered over, thanks to the setToolTip()
method.
Tooltips are versatile in PyQt, and you can assign them to almost any widget. They’re commonly used to provide users with helpful hints and information.
import sys |
To learn more about PyQt and enhance your skills, consider checking the related course or other helpful resources. If you are new to Python PyQt, then I highly recommend this book.
Happy coding with PyQt! Download PyQt Examples