We’ll be covering while loop in this tutorial.
A while loop let you do repeated execution of one or more lines of code, until the boolean condition changes.
The code block inside the while loop (four spaces indention) will execute as long as the boolean condition in the while loop is True.
Related Course: Complete Python Programming Course & Exercises
While Loop syntax
While loops exist in many programming languages, it repeats code.
Python’s while loop has this syntax:
while <expr>: |
<statements>
are one or more lines of code, they must be indented with four spaces.
The statements repeat until the expression changes.
The expression <exp>
is evaluated in Boolean context.
If the expression
<expr>
is True, the loops body is executed.While it stays True, it keeps repeating.
If the expression
<expr>
becomes False, it ends the loop.
Python while loop
Lets build a program that has a while
loop:
i = 1 |
This is what happens:
the expression
<expr>
isi < 6
, the code below repeats while True.it has two statements.
1st statement
print(f"i = {i}")
outputs variablei
.2nd statement
i = i + 1
increases i.the while loop stops when the
<expr>
is False:i >= 6
.
Lets have a look:
The program outputs:
i = 1 |
So why is 6 not included?
Because if a is equal to 6, the condition i < 6
is False.
To include six, change it to <=
.
You can make a countdown timer with a while loop:
i = 10 |
This will repeat until variable i is equal to one.
Related Course: Complete Python Programming Course & Exercises
While loop flow chart
The flow chart below shows the while loop:
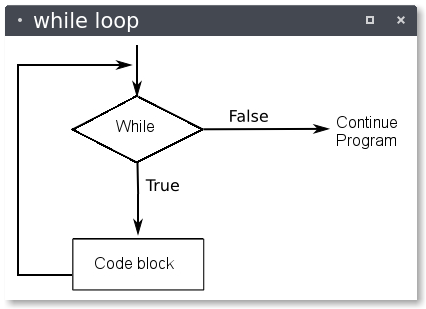
If the boolean condition of a while
loop is never changes, it could cause the program to run forever or to freeze/crash.
To make an infinite while loop, run the code below:
while True: |
A while loop terminates if the expression is False.
You can end execution by pressing Ctrl-Z
in the Python shell.
Break and continue
The statements break and continue modify the loops behavior.
the break statement exits the loop.
the continue statement skips the current cycle.
The program below uses the break statement:
1 n = |
break terminates the loop.
The example below shows the continue statement:
1 n = |
It skipped the third iteration because continue was used.
The continue statement skips one or more cycles.
The else clause
The else clause can be used with a while loop.
This is unique to Python.
The syntax is:
while <expr>: |
The <other_statement(s)
will only be executed in normal execution flow.
If the while loop ends because of the break keyword, it won’t be run.
The eample below does normal execution:
1 n = |
If break is called before ending the while loop, the else clause isn’t run.
1 n = |
That’s because the while loop is terminated prematurely with break.
Related Course: Complete Python Programming Course & Exercises
One-Line while loops
While loop can be one a single line, where multiple statements are separated by a semicolon ;
.
1 n = |
This behaves exactly as the multiline loop, it outputs:
|
But this only works for very simple while loops. You can’t add if statements inside.
PEP 8 discourages multiple statements on a single line.
Python while loop input
You can get a number from the keyboard using this line:
x = int(input("Guess a number:")) |
It gets keyboard input with the input() function that returns text.
That text is then convert to a number with int()
.
while x != 5: |
The above expression x != 5
will repeat until x is equal to five.
Then it keeps asking the user to input the number and only quits the loop when x is equal ==
to five.
x = 0
while x != 5:
x = int(input("Guess a number:"))
if x != 5:
print("Incorrect choice")
print("Correct")
This is defined in the line: while x != 5
, or in English, “while x is not equal to five, repeat”.
The program keeps repeating the code block while the condition (x != 5)
is True.
If x is equal to five, the program ends the loop.
Conclusion
In this tutorial, you learned about while loops.
- a while loop is indefinite iteration
- a while loop repeats code while the expression is True
- it exits the loop if the expression is False
- the break and continue statements change the loop behavior
If you are a Python beginner, then I highly recommend this book.
Ok.....I give.....what terminates the WHILE LOOP? I'm not asking when the loop condition changes, what terminates the code contained in the WHILE loop? Is it simply formatting? (TAB positions)
Without the condition being true, it will continue forever (or until memory overflow). The break keyword can make it stop as well. In most of the cases, you want to be sure the while loop condition is met. Some while loops should last forever except if the machine is turned of, like a web server or computer game.