In Python you can split a string with the split()
method. It breaks up a string (based on the given separator) and returns a list of strings.
To split a string, we use the method .split()
. This method will return one or more new strings. All substrings are returned in a newly created list.
Related course: Complete Python Programming Course & Exercises
Introduction
The split function breaks a string down into smaller chunks (a list of strings). Splitting a string is the opposite of concatenation (merges multiple strings into one string).
The split() method takes maximum of 2 parameters, of which one is optional. The syntax of the split() function is:
str.split([separator [, maxsplit]]) |
The first parameter seperator
is the string to split on, this could be a space, a comma, a dash, a word etcetera.
The second parameter maxsplit
is the maximum number of splits. By default there is no maximum and most programs don’t require a maximum number of splits.
String split example
We can split a string based on a character " "
, this is named the seperator.s = "To convert the result to"
parts = s.split(" ")
print(parts)
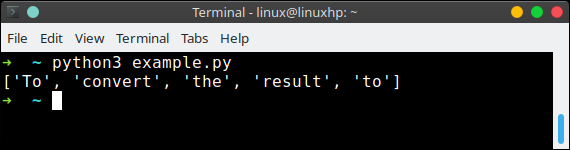
Any character (seperator) can be used. If you want to get sentences you could use:s = "Python string example. We split it using the dot character."
parts = s.split(".")
print(parts)
['Python string example', ' We split it using the dot character', '']
It’s not limited to a space, you can seperate on a comma:
"alice,allison,chicago,usa,accountant" s = |
You can split on a dot (turning texts into individual sentences)
"This is an example sentence. It has several words, and is in the English language. It's dummy data for Python." s = |
The seperator can be a word instead of a single symbol or character
s = s.split("is") |
Note that we overwrite s, when calling the s.split() function. This is optional, if you want to keep the string you can store the result in a new variable.
Something likewords = s.split()
If no separator is defined if you call the split() function, it will use a whitespace by default.
"hello world how are you" s = |
Related course: Complete Python Programming Course & Exercises