Strings in Python can be defined using quote symbols. An example of a string definition and output below:s = "Hello World"
print(s)
This will output to the terminal:
Related Course: Complete Python Programming Course & Exercises
Accesing array elements
You may access character elements of a string using the brackets symbol, which are [ and ]. We do so by specifying the string name and the index.
The example below prints the first element of a string.
print(s[0]) |
To print the second character you would write:print(s[1])
Related Course: Complete Python Programming Course & Exercises
String Slicing
You can slice the string into smaller strings. To do so you need to specify either a starting, ending index or both. Let us illustrate that in the Python shell:
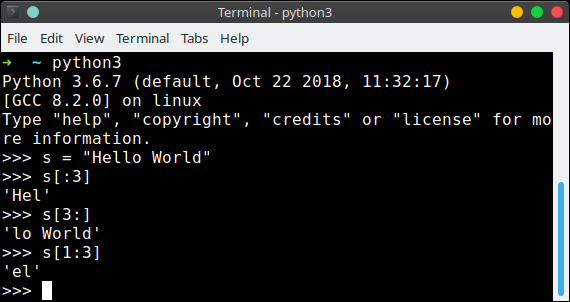
If no number is given, such as in s[:3]
it will simply take the beginning or end of teh string. We have accessed the string as if it was an array.
If you want to output them from your program, you have to wrap them in the print command. You can store the sliced string as a new string:slice = s[0:5]
Download exercises
"teh string"
I laughed when I saw that XD
So substrings are just specified like parts of a list?
Can you do it with lists too, like
?
Yes, they are specified like parts. It works with lists too. Both of them can be manipulated using the same style.