The zip()
function is an intrinsic utility in Python, instrumental for amalgamating two collections, given they share the same length. Collections in Python, such as lists, tuples, and dictionaries, serve as the core structures to store and handle data. In this guide, we will delve into the functionality of the zip()
function using various collections, enriched by illustrative Python 3 examples.
Diving Deep into Python Collections and the zip() Function
Python collections, encompassing structures like lists, tuples, and dictionaries, form the bedrock for data storage and manipulation. The zip()
function emerges as a potent method to combine these collections seamlessly when they are of equivalent length.
Comprehensive Guide to Zipping Lists in Python
Envision two lists dubbed list a and list b. For the sake of this demonstration, the range function helps us generate these lists, though manual list definitions are equally viable.
a = list(range(8)) |
The contents of both lists are as follows:
[0, 1, 2, 3, 4, 5, 6, 7] |
Using the zip()
function, we can unify these lists into a single cohesive list:
ab = list(zip(a,b)) |
The amalgamated list, termed ab
, is illustrated below:
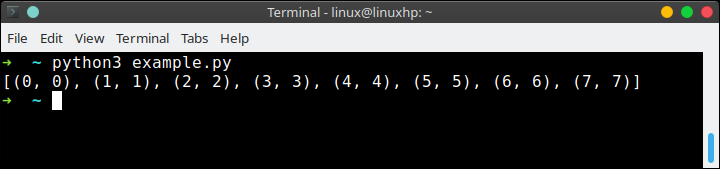
An observation reveals that each unit in the merged list is a tuple. Should we decide to merge three lists via zip()
, each unit would be a trio.
Key Takeaway: The act of merging two lists with zip()
ensures the resultant list has the same number of units as the original ones. To elucidate, zipping two 8-item lists culminates in a unified list comprising 8 tuples.
Converting Zipped Lists into Dictionaries
Moreover, the zip()
function can be harnessed to fabricate dictionaries. To achieve this, two separate lists - one carrying the keys and the other bearing values - can be integrated:
|
The fabricated dictionary will appear as:
{'Hawaii': 'Aloha', 'China': 'Nihao'} |
In encapsulation, the zip()
function stands out as a dynamic instrument in Python’s toolkit, facilitating streamlined merging of collections. Be it fusing lists or architecting dictionaries, zip()
proffers an elegant avenue to meet your data organization objectives.